Python args and kwargs

The
*args
and **kwargs
allow you to pass multiple arguments or keyword arguments to a
function. We can
pass a varying number of arguments to a function. We can simply pass
a list or a set of all the arguments to your function. But whenever
we call the function we’ll also need to create a list of arguments
to pass to it. This can be inconvenient, especially if we don’t
know up front all the values that should go into the list. The *args
can be really useful, because it allows you to pass a varying number
of positional arguments to your script. The *
is
called unpacking operator .The iterable object we’ll get used the
unpacking operator *
is not a list but is a tuple. Both the tuple and list support
slicing and iteration. In fact, tuples are very different in at least
one aspect, i.e., lists are mutable while tuples are not.
Example for *args :
def myfun(
*
argv):
for arg in argv:
print (arg)
myfun('Hello', 'Welcome', 'to', 'python')
Output :
Hello
Welcome
to
python
The **kwargs
works just args
Example
for
*kwargs
:
def myfun(
**
kwargs):
d={}
for key,value in kwargs.items():
d[key]=value
print(d)
myfun(first ='welcome', mid ='to’, last='python')
output:
{'mid': 'to', 'last': 'python', 'first': 'welcome'}
The double asterisk form of
**kwargs
is used to pass a key worded, variable-length argument dictionary to a function.
Like non-default arguments have to precede default arguments, *args
**kwargs
The correct order for your parameters is:
- Standard arguments.
*
args
arguments.**
kwargs
arguments.
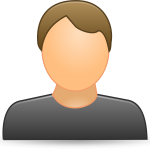